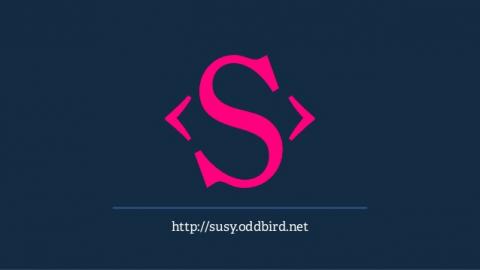
Although I like Bootstrap and Foundation I prefer to avoid writing classes like class="col-md-12" or class="row". I discovered Susy and I am in love with it. Susy provides all we need to create custom layouts and grids with lot of flexibility.
Getting started
There are different ways to install Susy, if you are using Bower to manage your dependencies, you can install it with this command:
bower install susy --save
Another way is with RubyGems:
gem install susy
If you are using Grunt, you have to install grunt sass package, and then, require susy in the task option.
// Gruntfile.js
sass: {
dist: {
options: {
style: 'expanded',
require: 'susy'
},
files: {
'css/style.css': 'scss/style.scss'
}
}
}
When you have all installed, you only need to import susy in your project.
@import "susy";
Basic layout
Suppose that you need to create a basic layout: A main content with a right sidebar. If you are using Bootstrap, you need to write an HTML code like this:
<div class="wrap">
<div class="content col-md-4" id="sidebar"></div>
<div class="content col-md-8" id="content"></div>
</div>
The problem is that Bootstrap and Foundation come with lot of baggage. Also you do not need all the stuff they give. With Susy you can create the content from scratch like this:
<div class="wrap">
<div class="sidebar"></div>
<div class="content"></div>
</div>
Before start with Susy, you need to set some settings. All of this settings are described here. To start we need to know three settings.
@import "susy"$susy: (
columns: 12,
container: 1120px,
global-box-sizing: border-box
);
@include border-box-sizing;
The columns property is the total of columns of your grid. Feel free to change it with another value. The container property sets the size of the layout.
Finally, the global-box-sizing sets box-sizing: border-box to all html tags.
I recommend the container mixin to create the container for your website. In the example above, it's the wrap class:
.wrap {
@include container;
}
Finally, to set the size of the content and sidebar, we can tell Susy how many columns they have:
.sidebar {
@include span(4);
}.content {
@include span(8 last);
}
We do have to let Susy know that content is the last item on the row. So we need to add last on the span mixin.
Check this demo to see the results.
Create responsive grids
The next step is to make this grid responsive. Breakpoint provides us a very easy way to create media queries with Sass. Install it with Bower with this command:
bower install breakpoint-sass --save
Then, import breakpoint in your project:
//your scss file
@import 'breakpoint';
@import 'susy';
The last step is to define variables with the value of your media queries. By defaul Breakpoint assumes that your media query is with min-width if you pass only a number:
$large: 1600px; //min-width
$middle: 800px 1024; //sets min-width and max-width
$small: max-width 420px;
$custom-orientation: (min-height 1000px) (orientation portrait);
For example, if you need to change some value for mobile screen, you have to do that:
.container {
width: 50%;
float: left; @include breakpoint($small) {
width: 100%;
float: none;
}
}
Very easy!
Adapting the last example for devices
Let's create a very basic responsive layout for the last example. In this case, we put the sidebar in the first position, and then the content.
Add a variable which represents the smartphone media query:
$mobile: max-width 480px;
Then, add the media queries in your grid configuration to get the full size for mobile devices.
.sidebar {
@include span(3);
border: solid 1px #D9D9D9;
height: 200px;
@include breakpoint($mobile) {
@include span(12);
}
}.content {
@include span(9 last);
border: solid 1px #D9D9D9;
height: 200px;
@include breakpoint($mobile) {
@include span(12);
}
}
See this pen to check the final result. Do not forget to resize the browser!
Conclusion
Susy is a very powerful tool to create custom grids. With Breakpoint you can create media queries easily. As I said, I like Bootstrap and I like working with it, but I prefer to avoid its classes and all of its code. I like clean grids and semantic HTML, Susy helps me to get that and I become Susy fan :)